728x90
Board.js
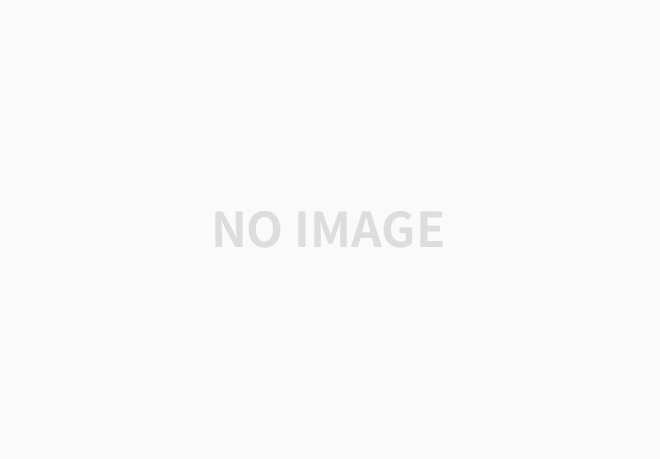
import React, { useState } from 'react';
import './Board.css';
// 더미 데이터를 위한 임시 배열
const initialPosts = [
{ id: 1, title: '첫 번째 게시글', content: '이것은 첫 번째 게시글의 내용입니다.' },
{ id: 2, title: '두 번째 게시글', content: '이것은 두 번째 게시글의 내용입니다.' }
];
const Board = () => {
const [posts, setPosts] = useState(initialPosts);
const [newPost, setNewPost] = useState({ title: '', content: '' });
// 게시글 추가 핸들러
const handleAddPost = () => {
if (newPost.title && newPost.content) {
setPosts([...posts, { id: posts.length + 1, ...newPost }]);
setNewPost({ title: '', content: '' });
}
};
// 입력 값 변경 핸들러
const handleChange = (e) => {
const { name, value } = e.target;
setNewPost({ ...newPost, [name]: value });
};
return (
<div className="board">
<h1>게시판</h1>
{/* 게시글 목록 */}
<div className="posts">
{posts.map((post) => (
<div key={post.id} className="post">
<h2>{post.title}</h2>
<p>{post.content}</p>
</div>
))}
</div>
{/* 게시글 추가 폼 */}
<div className="add-post">
<h2>새 게시글 추가</h2>
<input
type="text"
name="title"
placeholder="제목"
value={newPost.title}
onChange={handleChange}
/>
<textarea
name="content"
placeholder="내용"
value={newPost.content}
onChange={handleChange}
/>
<button onClick={handleAddPost}>추가</button>
</div>
</div>
);
};
export default Board;
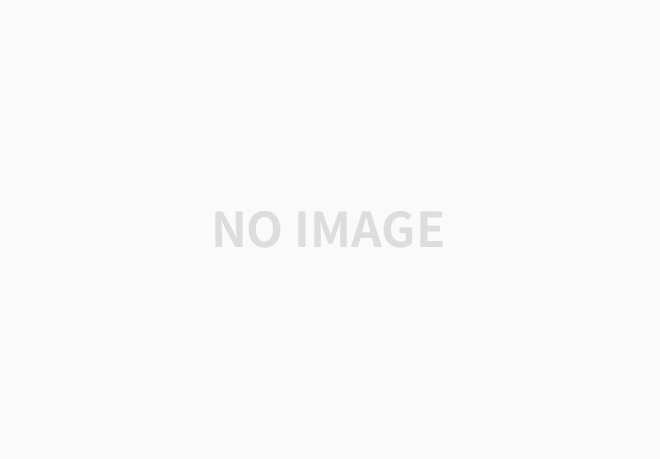
리엑트에서 간단하게 구현한 결과
제목과 내용을 누르면 추가가 된다.
728x90
'주니어 기초 코딩공부 > React 기초' 카테고리의 다른 글
[React] 기초 App.tsx > npm start (0) | 2024.09.23 |
---|---|
[React] 초기 Board.css (0) | 2024.09.23 |
[React] 처음 리엑트를 하라고 하셨다... Node.js 및 npm 설치하기 (2) | 2024.09.22 |
리액트 기초 입문 (맥북 프로 초기 설정) (0) | 2023.04.25 |
리액트 실무 - Nopde.js, Express 수업 내용 (0) | 2023.04.11 |